WordPress でカスタム投稿とカスタムフィールドを追加してみた。
2024年3月10日
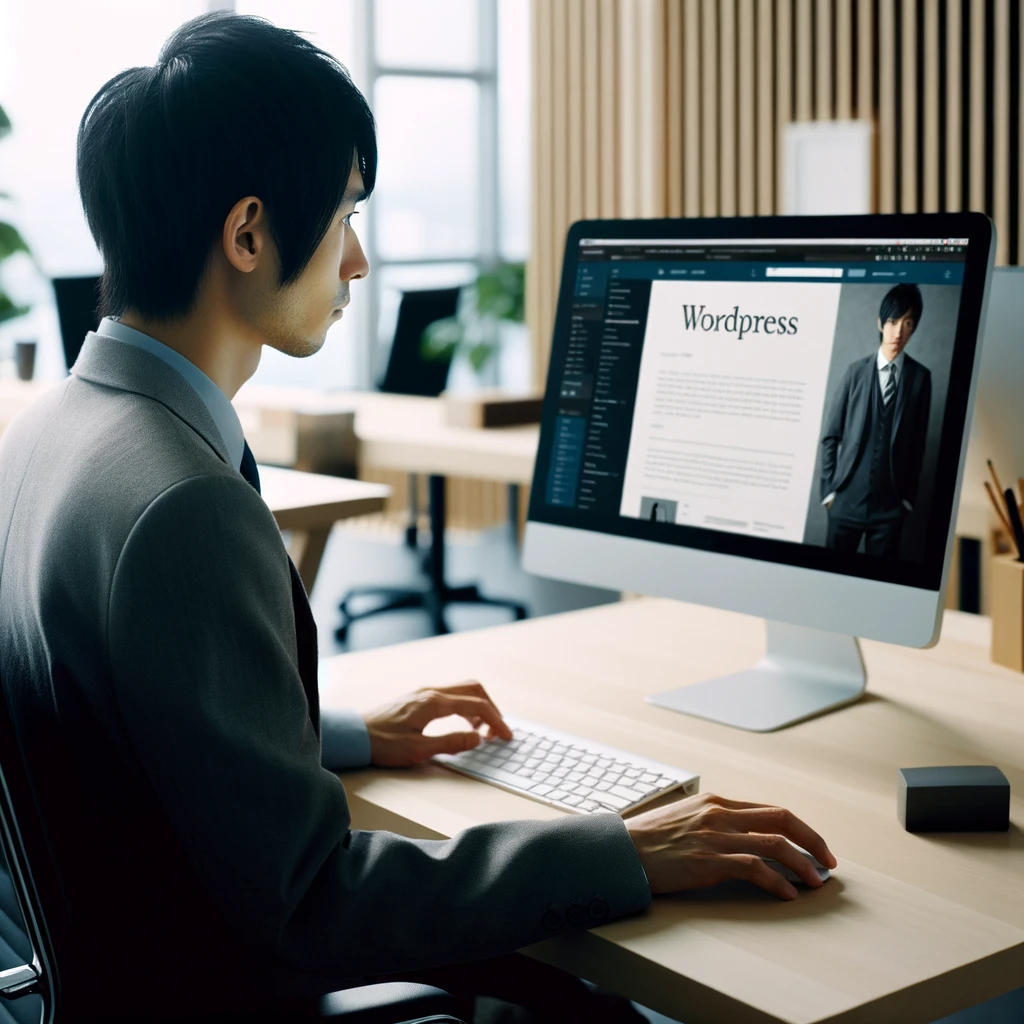
WordPress でオリジナルのコンテンツを配信していると、定型的な項目を追加したくなることがあります。
今回のゴール
今回は「書籍紹介」というオリジナルの投稿タイプやカテゴリーを追加し、そこに「著者」や「出版年」といったオリジナル項目を追加していきます。
カスタム投稿タイプとカテゴリーの追加
オリジナルの投稿タイプとカテゴリーを追加するには functions.php を編集します。
今回は以下の様なコードを functions.php に追加します。
add_action('init', 'create_post_type');
function create_post_type() {
register_post_type(
'book',
array(
'label' => '書籍紹介',
'public' => true,
'has_archive' => true,
'menu_position' => 5,
'show_in_rest' => true,
'supports' => array(
)
)
);
register_taxonomy(
'book-cat',
'book',
array(
'label' => '書籍カテゴリー',
'hierarchical' => true,
'public' => true,
'show_in_rest' => true
)
);
ここでは WordPress が用意している register_post_type 関数と register_taxonomy 関数を呼び出しています。register_post_type がオリジナルの投稿タイプの追加、register_taxonomy がそのカテゴリーを追加しています。
WordPress が用意している関数については WordPress がリファレンスを公開しているので、そちらを参考にすると良いでしょう。(英語)
https://developer.wordpress.org/reference/
register_post_type と register_taxonomy については以下のページで確認できます。
https://developer.wordpress.org/reference/functions/register_post_type/
https://developer.wordpress.org/reference/functions/register_taxonomy/
上記のコードが正常に動くと、WordPress のメニューに「書籍紹介」や「書籍カテゴリー」の項目が追加されます。
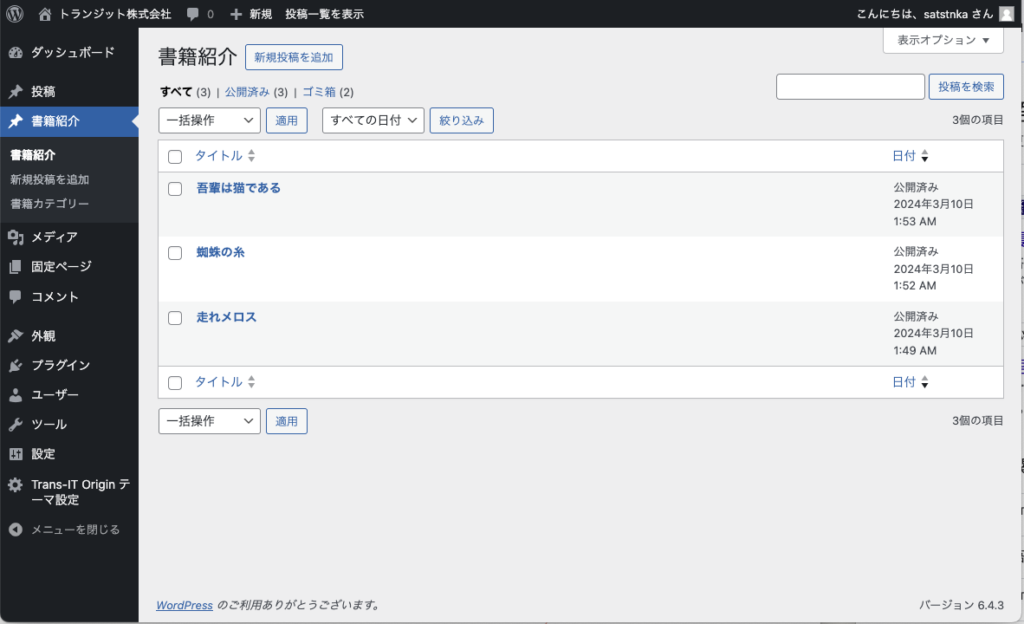
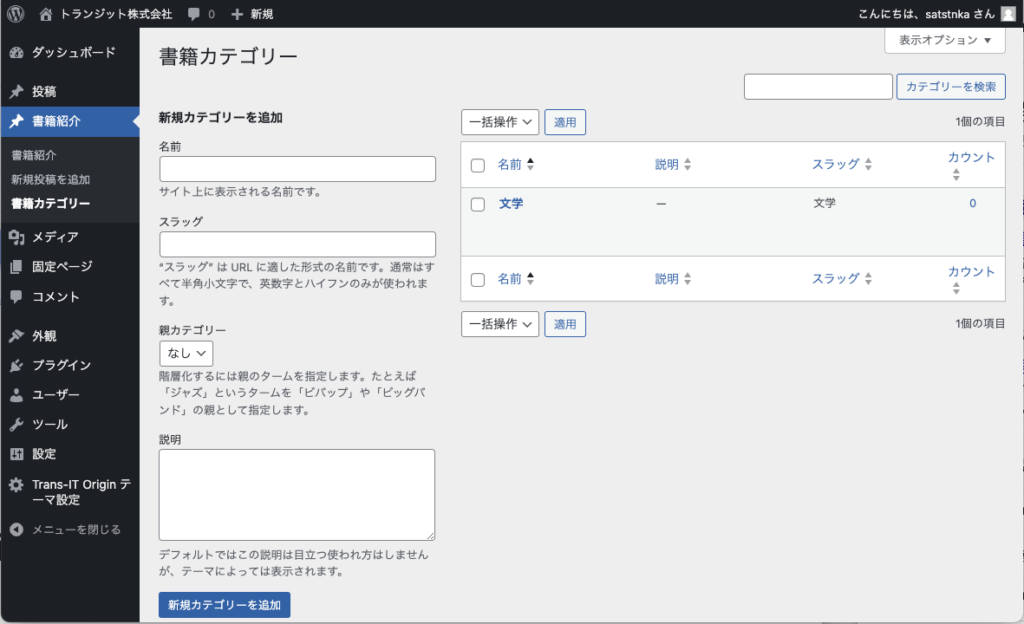
カスタムフィールドの追加
続いて、「書籍紹介」の投稿時に入力する「著者」や「出版年」の項目を追加します。こちらも functions.php を修正して以下のコードを追加していきます。
add_action('add_meta_boxes', 'create_meta_box');
add_action('save_post', 'save_additional_parameters');
function create_meta_box() {
add_meta_box(
'book_author',
'著者',
'show_book_author',
'book',
'normal',
'high'
);
add_meta_box(
'book_published_year',
'出版年',
'show_book_published_year',
'book',
'normal',
'high'
);
}
function show_book_author() {
global $post;
$author = get_post_meta($post->ID, 'book_author', true);
echo '<input type="text" name="book_author" value="' . esc_attr($author) . '" />';
}
function show_book_published_year() {
global $post;
$published_year = get_post_meta($post->ID, 'book_published_year', true);
echo '<input type="text" name="book_published_year" value="' . esc_attr($published_year) . '" />';
}
function save_additional_parameters($post_id) {
if(array_key_exists('book_author', $_POST)) {
update_post_meta($post_id, 'book_author', $_POST['book_author']);
}
if(array_key_exists('book_published_year', $_POST)) {
update_post_meta($post_id, 'book_published_year', $_POST['book_published_year']);
}
}
まず、create_meta_box 関数が、カスタムフィールドの表示、save_additional_parameters 関数がカスタムフィールドの保存部分になります。
create_meta_box の中では、WordPress で用意されている add_meta_box を呼び出し、書籍や出版年といったオリジナルの項目を追加しています。また、show_book_author, show_book_published_year 関数はそのフィールドを表示する部分になります。
https://developer.wordpress.org/reference/functions/add_meta_box/
save_additional_parameters の中では WordPress で用意されている update_post_meta 関数を呼び出し、ユーザーが入力した内容を保存しています。
https://developer.wordpress.org/reference/functions/update_post_meta/
正常に動作すれば、書籍紹介で新規投稿をしたときに「著者」や「出版年」の項目が表示されていることが確認できると思います。
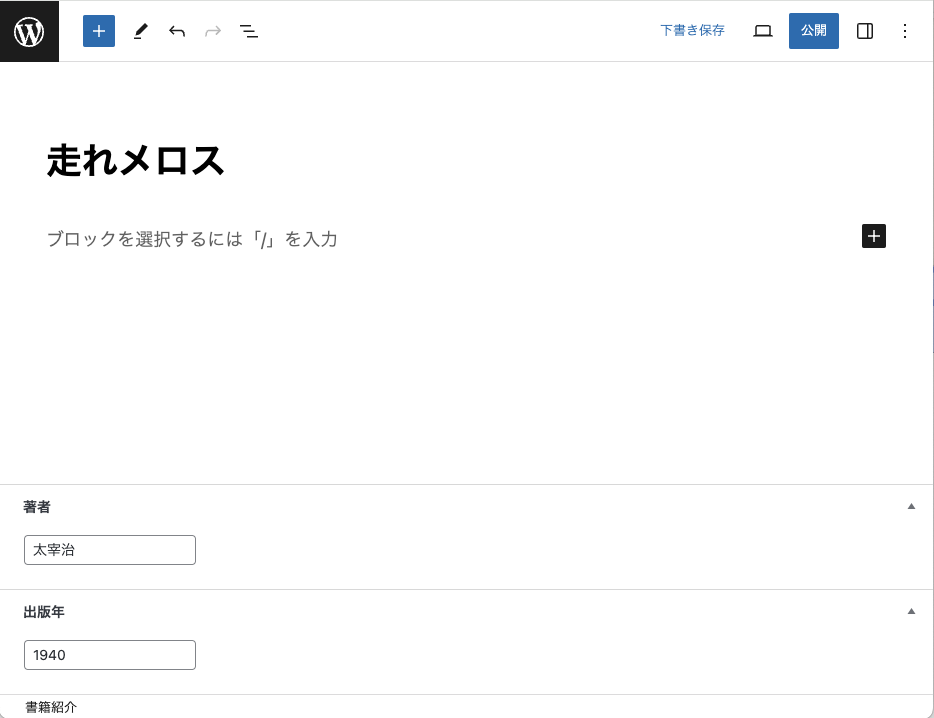
カスタムフィールドを表示する。
最後に実際にユーザーが入力したカスタムフィールドを表示したいと思います。今回は “book” という名前でカスタム投稿を登録しているので一覧表示であれば archive-book.php、投稿ページであれば single-book.php で表示していきます。
今回の例では一覧を表示する archive-book.php の例を書いてみたいと思います。
<?php if(have_posts()): ?>
<table>
<tr>
<th>書籍名</th>
<th>著者</th>
<th>出版年</th>
</tr>
<?php while(have_posts()): the_post(); ?>
<tr>
<td>
<a href="<?php the_permalink(); ?>">
<?php the_title(); ?>
</a>
</td>
<td><?php echo get_post_meta($post->ID, 'book_author', true); ?></td>
<td><?php echo get_post_meta($post->ID, 'book_published_year', true); ?></td>
</tr>
<?php endwhile; ?>
<?php endif; ?>
入力されたカスタムフィールドの取得には WordPress が用意した関数 get_post_meta を使用します。
https://developer.wordpress.org/reference/functions/get_post_meta/
書籍紹介の投稿一覧の画面最上部に「投稿一覧を表示」というメニューがあるので、そちらをクリックするとタイトル、著者、出版年の一覧が表示されると思います。
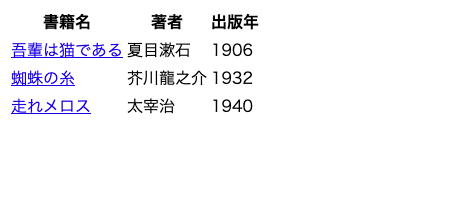
まとめ
以上、WordPress でオリジナルの投稿タイプとフィールドを追加する方法を紹介してみました。
PHP の知識が多少必要ですが、今回の書籍紹介以外にも観光地紹介、飲食店紹介、ゲーム紹介、商品紹介など色々なケースで活用できそうですね。